CopyCat - Make all your Raspberry Pis show the same colour
We recommend using the new driver free based scripts for LedBorg.
The new driver free examples can be found here, the installation can be found here.
So you have two or more LedBorgs, but you want them to always show the same colour, then CopyCat is the script for you.
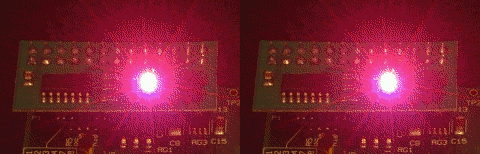
CopyCat is a script that comes in two parts:
- CopyCatS.py (Server)
This script runs on one of the Raspberry Pis and sends out what LedBorg colour is currently set at a regular interval - CopyCatC.py (Client)
This script runs on all other Raspberry Pis which you want to copy the colour to, which set the LedBorg colour when updated
broadcastIP
in CopyCatS.py, line 10
IP address to send to, may be a single machine (e.g. 192.168.1.5) or a broadcast (e.g. 192.168.1.255) where '255' is used to indicate that number is everybodybroadcastPort
in CopyCatS.py, line 11
Number used to identify who gets network messages, if two copies of CopyCatS.py are used in the same network this should be changed to identify which copy is whichinterval
in CopyCatS.py, line 12
Delay between updates, smaller numbers update faster but send more messagesportListen
in CopyCatC.py, line 9
If you change the port your CopyCatS.py is using, change this to match
and you can download the CopyCatC script file as text here.
Save the text files on your pi as CopyCatS.py and CopyCatC.py respectively.
Make the scripts executable using
chmod +x CopyCat*.py
and run on the sending Raspberry Pi using
./CopyCatS.py
and run on the receiving Raspberry Pis using
./CopyCatC.py
CopyCatS
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import socket import sys import time # Settings for the CopyCat Server broadcastIP = '192.168.0.255' # IP address to send to, 255 in one or more positions is a broadcast / wild-card broadcastPort = 9037 # What message number to send with (LEDb on an LCD) interval = 0.1 # Number of seconds between updates, smaller responds quicker but uses more resources # Setup the connection for sending on sender = socket.socket(socket.AF_INET, socket.SOCK_DGRAM, socket.IPPROTO_UDP) # Create the socket sender.setsockopt(socket.SOL_SOCKET, socket.SO_BROADCAST, 1) # Enable broadcasting (sending to many IPs based on wild-cards) sender.bind(('0.0.0.0', 0)) # Set the IP and port number to use locally, IP 0.0.0.0 means all connections and port 0 means assign a number for us (do not care) # Function to read the LedBorg colour def GetLedColour(): LedBorg = open('/dev/ledborg', 'r') # Open the LedBorg device for reading from colour = LedBorg.read() # Read the colour string from the LedBorg device LedBorg.close() # Close the LedBorg device return colour # Return the read colour # Loop indefinitely while True: # Get the colour of the local LedBorg colour = GetLedColour() # Send the colour to others sender.sendto(colour, (broadcastIP, broadcastPort)) # Wait for interval time.sleep(interval)
CopyCatC
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import SocketServer import sys # Settings for the CopyCat client portListen = 9037 # What messages to listen for (LEDb on an LCD) # Class used to handle UDP messages class LedBorgHandler(SocketServer.BaseRequestHandler): # Function called when a new message has been received def handle(self): request, socket = self.request # Read who spoke to us and what they said self.SetLedColour(request) # Set the colour to what they said # Function to write the LedBorg colour def SetLedColour(self, colour): LedBorg = open('/dev/ledborg', 'w') # Open the LedBorg device for writing to LedBorg.write(colour) # Write the colour string to the LedBorg device LedBorg.close() # Close the LedBorg device # Setup the UDP listener copyCatClient = SocketServer.UDPServer(('', portListen), LedBorgHandler) # Loop indefinitely while True: copyCatClient.handle_request()
