Sorry, couple noob questions.... What Do I need..?
Forums:
Hi,
Trying to do a little set up, I have the Pi, I have the Ultraborg Board, ultrasonic and a Vibe motor. My question is this... Do I require any other boards to complete this set up?
What I am trying to do is this, when someone walks past the Pi the vibe motor starts about 10 seconds later for about 15 seconds.
So can anyone advise me please, have i missed any components needed for this? And also looking at the guides, which set up would be best for the Vibe motor as all the other set ups seem to be for a servo.
Please be gentle, VERY VERY new at all this and brain feels like its about to explode lol

piborg
Fri, 03/04/2016 - 11:50
Permalink
Vibe motor driver
You have most of what you need, but you will need something to drive the vibe motor.
Basically the Raspberry Pi GPIO cannot provide enough power to drive a motor.
This means you will need a board or circuit which can provide the power but allows the Raspberry Pi to control the motor output.
UltraBorg has outputs which are intended to work with RC car style servos.
These connect a little differently to the normal DC motors which work the same way as your vibe motor will.
Unfortunately the two require different connections and are not compatible.
Put simply the UltraBorg is not capable of powering the vibe motor.
If this is a small vibe motor than something like our PicoBorg may be strong enough to provide the power.
If the vibe motor needs more than 1.0 A of current you will want something a bit more powerful, like a PicoBorg Reverse.
The only other thing you will need is some power for the vibe motor.
Most drive boards have a minimum voltage requirement, also the vibe motor will have an intended voltage.
You will want a power supply or set of batteries which is at least the larger of these two voltages.
For example if you have a 6V vibe motor that uses 0.5 A then PicoBorg would be capable of driving it correctly.
PicoBorg will work with any voltage upto 12V and the motor needs 6V, so you would want a 6V supply.
Using rechargeable AAs (1.2V) you would need 5 batteries, you could use 6 though.
Hopefully this all makes sense, I know motors can be a bit of a confusing topic.
lars_the_bear
Fri, 03/04/2016 - 15:23
Permalink
Hi
Hi
If you don't mind a bit of DIY, you could do it this way:
http://bildr.org/2012/03/rfp30n06le-arduino/
The components only cost of couple of quid, and it's educational and fun :)
Of course, if you're not a tinkerer and just want something that will slot together, I can see the appeal of a ready-made board.
Best wishes
Kevin
djwmcnae82@gmail.com
Tue, 03/29/2016 - 09:41
Permalink
Thanks for the replies, sorry
Thanks for the replies, sorry I didnt get an email saying you had replied :o)
The vibe motor I have was purchased from here :o)
Thanks again for your help, the next question is the coding!
What I am trying to achieve is motion is detected and about 5-10 seconds later the vibe motor starts for about 3-5 seconds. Any help on this part would be great as well please.
piborg
Tue, 03/29/2016 - 10:48
Permalink
Simple example
Here is a simple example in Python which should get you started.
It checks for a small distance reading, if it sees one it waits and then runs the vibe motor.
The code is setup for driving the GPIO pin connected to drive #1 on the PicoBorg.
djwmcnae82@gmail.com
Tue, 03/29/2016 - 11:09
Permalink
Thank you!
Thanks for your help, and I am sorry for being a complete noob at this, loving the learning part though!
So, I have the pi, the vibe motor and the above script. If I use PicoBorg Reverse i can use this to add power to it as well as control the vibe, is that correct? Are there any files that show me how to set it all up... IE the hardware side of things.
piborg
Tue, 03/29/2016 - 11:34
Permalink
Vibe motr with PicoBorg Reverse
Yes, with some changes to the script a PicoBorg Reverse will be able to power the vibe motor for you.
This should be the correct wiring diagram for what you need:
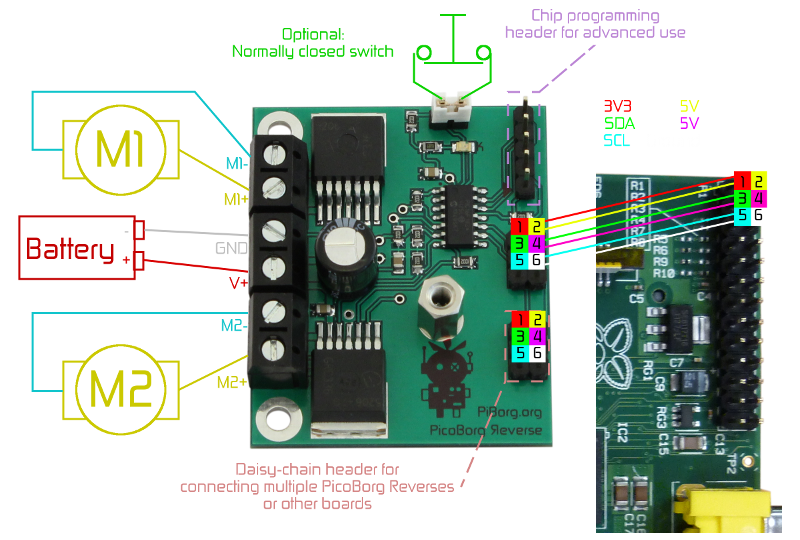
Connect the vibe motor as M1, you do not need to connect a second motor.
Ignore the switch and just leave the jumper attached to the board.
For the battery you will need at least 6V to get PicoBorg Reverse to work.
You should connect the 3-pin cables so that the PicoBorg Reverse is connected to the daisy-chain connector on the UltraBorg.
See this forum post for more detail: https://www.piborg.org/node/1833
Here is the same example adjusted to use PicoBorg Reverse:
You will want to change the
voltageIn
value to the battery voltage attached to the PicoBorg Reverse.djwmcnae82@gmail.com
Tue, 03/29/2016 - 16:34
Permalink
Thanks
Ok, will need get the parts ordered and take on the challenge!! Thanks again.
djwmcnae82@gmail.com
Tue, 03/29/2016 - 16:40
Permalink
Stock
DO You know When will be back in Stock?
piborg
Tue, 03/29/2016 - 17:33
Permalink
PicoBorg Reverse stock
We currently have PicoBorg Reverses in production, they should be available within a week or two.