UpdateLed - Use your LedBorg to check for updates on your Raspberry Pi
We recommend using the new driver free based scripts for LedBorg.
The new driver free examples can be found here, the installation can be found here.
So you have your Raspberry Pi server running headless, how can you check if there are any updates for it without using SSH or VNC?
Never fear, UpdateLed will save you!
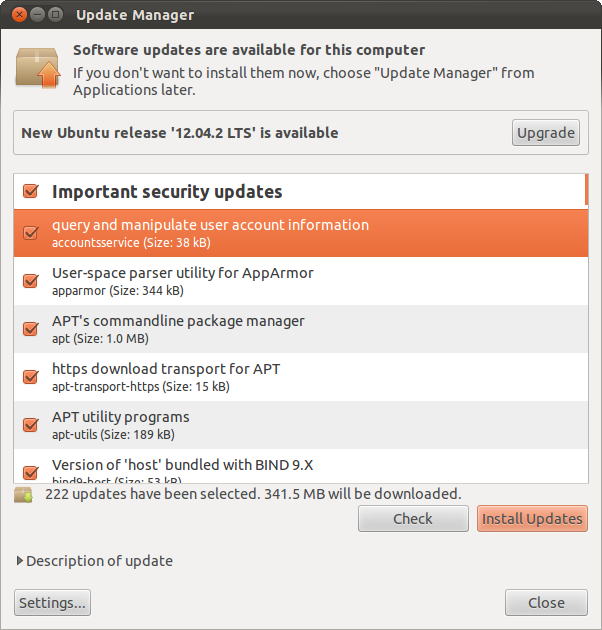
UpdateLed takes your LedBorg and turns it into a status indicator showing how many updates are available!
In the standard configuration it will tell you how many updates are waiting with the following chart:
None | 1 to 9 | 10 to 24 | 25 to 49 | 50 or more | Failed to check |
Options include:
- updateColours, lines 11-17
Sets what quantity of updates show what colours - showWorking, line 23
True to show a different colour when busy - workingColour, line 20
Colour to use when busy if showWorking is True - errorColour, line 21
Colour to use if an update count cannot be worked out - interval, line 22
Number of minutes delay between checks, too small will cause a lot of network traffic
So how many updates did our test rig have:
A nice round 10 :)
Here's the code, you can download the UpdateLed script file as text here
Save the text file on your pi as UpdateLed.py
Make the script executable using
chmod +x UpdateLed.py
and run using
./UpdateLed.py
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import os import re import time # Colour mapping for update count, ordered from largest to smallest # [minimum updates, colour code] updateColours = [ [50, '200'], # Lots of updates [25, '210'], # A fair number of updates [10, '220'], # Some updates [1, '120'], # At least one update [0, '020'], # No updates ] # Other settings workingColour = '002' # Colour to use whilst checking errorColour = '202' # Colour to use when we have had a problem interval = 60 # Number of minutes between checks showWorking = False # True to show workingColour when busy # Function to write the LedBorg colour def SetLedColour(colour): LedBorg = open('/dev/ledborg', 'w') # Open the LedBorg device for writing to LedBorg.write(colour) # Write the colour string to the LedBorg device LedBorg.close() # Close the LedBorg device # Loop indefinitely while True: # Set the working colour if showWorking: SetLedColour(workingColour) # Update the repository listing os.system('sudo apt-get update 2>&1 > /dev/null') # The '2>&1 > /dev/null' is used to suppress any messages # Query apt-get for the updates it would perform in simulation mode (does not need to be root) rawDataFile = os.popen('apt-get -s upgrade 2> /dev/null') # The '2> /dev/null' is used to suppress any errors rawData = rawDataFile.readlines() rawDataFile.close() # Parse data looking for the updates summary found = False for line in rawData: if re.match('\d+ upgraded, \d+ newly installed, \d+ to remove and \d+ not upgraded.', line): # We have found the right line, split the raw text into usable chunks found = True line = re.sub('[^\d]+', ' ', line) # Replace all non-numerics with a single space line = line.strip() # Remove any leading or trailing spaces token = line.split(' ') # Split by the spaces to get numbers # Convert all chunks to actual numbers for i in range(len(token)): token[i] = int(token[i]) # Skip checking any other lines break # Set the colour for the number of updates if found: numUpdates = token[0] print '%d updates available' % (numUpdates) # Search for the correct colour mapping and set it found = False for updateColour in updateColours: if numUpdates >= updateColour[0]: found = True SetLedColour(updateColour[1]) break if not found: print 'No colour mapping for %d updates...' % (numUpdates) SetLedColour('000') else: # We could not get any data, display an error print 'Unable to read update count!' SetLedColour(errorColour) # Wait for the interval (in minutes) time.sleep(interval * 60)
