TurtleBorgTypeR - TurtleBorg upgraded for PiCy Type R
So you built yourself a PiCy Type R following the ReverseKeyBorg instructions, but now the TurtleBorg script does not work, how do we fix it?
We need to upgarde TurtleBorg to TurtleBorgTypeR (^_^)
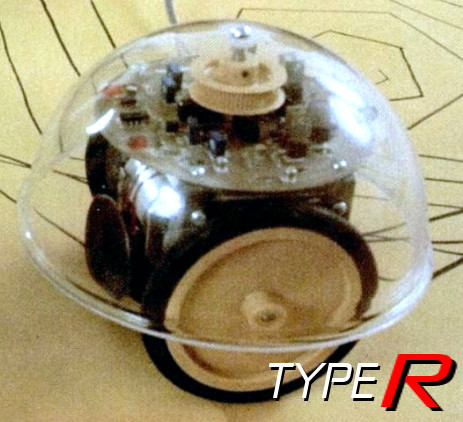
There are some slight differences with the commands, they are now:
The script has been designed so that it may be called directly from the terminal (or a Bash script) as well as being usable by importing it into another python script and calling the underlying functions.
The main script is TurtleBorgTypeR.py, a Python script which takes a command to execute or is imported into another Python script
Here's the code, you can download the TurtleBorgTypeR script file as text here
Save the text file on your pi as TurtleBorgTypeR.py
Make the script executable using
and run using
To get you started we have also put up a pair of examples in Bash and Python which both move the turtle in a square, you may need to change the time on the left turns to make it square on your robot!
The Bash example is here and should be saved as TurtleBorgTypeRTest.sh on your pi.
The Python example is here and should be saved as TurtleBorgTypeRTest.py on your pi.
Make the test scripts executable using
and run using
We need to upgarde TurtleBorg to TurtleBorgTypeR (^_^)
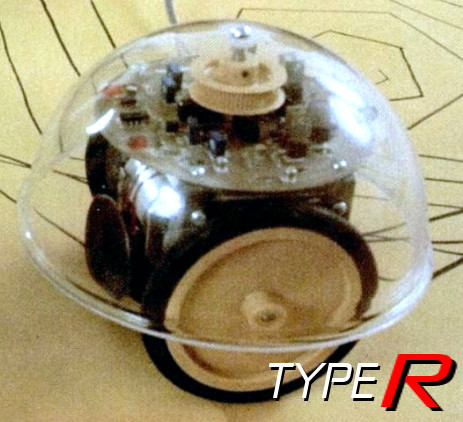
There are some slight differences with the commands, they are now:
FORWARD n
Moves forward for n seconds (shorthandFD
)LEFT n
Moves left for n seconds (shorthandLT
)RIGHT n
Moves right for n seconds (shorthandRT
)BACKWARD n
Moves backward for n seconds (shorthandBD
)HELP
Displays the commands available (shorthand?
)
FD 0.25
would move forward for a quarter of a second.The script has been designed so that it may be called directly from the terminal (or a Bash script) as well as being usable by importing it into another python script and calling the underlying functions.
The main script is TurtleBorgTypeR.py, a Python script which takes a command to execute or is imported into another Python script
Here's the code, you can download the TurtleBorgTypeR script file as text here
Save the text file on your pi as TurtleBorgTypeR.py
Make the script executable using
chmod +x TurtleBorgTypeR.py
and run using
sudo ./TurtleBorgTypeR.py command time_if_needed
To get you started we have also put up a pair of examples in Bash and Python which both move the turtle in a square, you may need to change the time on the left turns to make it square on your robot!
The Bash example is here and should be saved as TurtleBorgTypeRTest.sh on your pi.
The Python example is here and should be saved as TurtleBorgTypeRTest.py on your pi.
Make the test scripts executable using
chmod +x TurtleBorgTypeRTest.*
and run using
sudo ./TurtleBorgTypeRTest.py
sudo ./TurtleBorgTypeRTest.sh
TurtleBorgTypeR.py
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import sys import time import RPi.GPIO as GPIO GPIO.setmode(GPIO.BCM) GPIO.setwarnings(False) # Set which GPIO pins the drive outputs are connected to DRIVE_1 = 4 DRIVE_2 = 18 DRIVE_3 = 8 DRIVE_4 = 7 # Set all of the drive pins as output pins GPIO.setup(DRIVE_1, GPIO.OUT) GPIO.setup(DRIVE_2, GPIO.OUT) GPIO.setup(DRIVE_3, GPIO.OUT) GPIO.setup(DRIVE_4, GPIO.OUT) # Map of functions to drive pins leftDrivePos = DRIVE_1 # Drive number for left motor positive relay leftDriveNeg = DRIVE_2 # Drive number for left motor negative relay rightDrivePos = DRIVE_3 # Drive number for right motor positive relay rightDriveNeg = DRIVE_4 # Drive number for right motor negative relay # Functions for the robot to perform def MoveForward(n): """Move forward for 'n' seconds""" GPIO.output(leftDriveNeg, GPIO.LOW) GPIO.output(rightDriveNeg, GPIO.LOW) GPIO.output(leftDrivePos, GPIO.HIGH) GPIO.output(rightDrivePos, GPIO.HIGH) time.sleep(n) GPIO.output(leftDrivePos, GPIO.LOW) GPIO.output(rightDrivePos, GPIO.LOW) def MoveLeft(n): """Move left for 'n' seconds""" GPIO.output(leftDrivePos, GPIO.LOW) GPIO.output(rightDriveNeg, GPIO.LOW) GPIO.output(leftDriveNeg, GPIO.HIGH) GPIO.output(rightDrivePos, GPIO.HIGH) time.sleep(n) GPIO.output(leftDriveNeg, GPIO.LOW) GPIO.output(rightDrivePos, GPIO.LOW) def MoveRight(n): """Move right for 'n' seconds""" GPIO.output(leftDriveNeg, GPIO.LOW) GPIO.output(rightDrivePos, GPIO.LOW) GPIO.output(leftDrivePos, GPIO.HIGH) GPIO.output(rightDriveNeg, GPIO.HIGH) time.sleep(n) GPIO.output(leftDrivePos, GPIO.LOW) GPIO.output(rightDriveNeg, GPIO.LOW) def MoveBackward(n): """Move backward for 'n' seconds""" GPIO.output(leftDrivePos, GPIO.LOW) GPIO.output(rightDrivePos, GPIO.LOW) GPIO.output(leftDriveNeg, GPIO.HIGH) GPIO.output(rightDriveNeg, GPIO.HIGH) time.sleep(n) GPIO.output(leftDriveNeg, GPIO.LOW) GPIO.output(rightDriveNeg, GPIO.LOW) def HelpMessage(n): """Display a list of available commands""" print '' print 'Available commands:' commands = dCommands.keys() commands.sort() for command in commands: print '% 10s - %s, %s' % (command, dCommands[command].func_name, dCommands[command].__doc__) print '' # Map of command names to functions dCommands = { 'FORWARD':MoveForward, 'FD':MoveForward, 'LEFT':MoveLeft, 'LT':MoveLeft, 'RIGHT':MoveRight, 'RT':MoveRight, 'BACKWARD':MoveBackward, 'BD':MoveBackward, 'HELP':HelpMessage, '?':HelpMessage } # If we have been run directly then look at command line if __name__ == "__main__": # Process command if len(sys.argv) > 1: # Extract the command name and value (if there is any) command = sys.argv[1].upper() if len(sys.argv) > 2: sValue = sys.argv[2].upper() else: sValue = '0' try: fValue = float(sValue) except: fValue = 0.0 # Select the appropriate function and call it if dCommands.has_key(command): dCommands[command](fValue) else: print 'Command "%s" not recognised' % (command) HelpMessage(fValue) else: # No command, display the help message print 'Usage: %s command [n]' % (sys.argv[0]) HelpMessage(0)
TurtleBorgTypeRTest.sh
#!/bin/bash /home/pi/TurtleBorgTypeR.py fd 1 /home/pi/TurtleBorgTypeR.py lt 0.40 /home/pi/TurtleBorgTypeR.py fd 1 /home/pi/TurtleBorgTypeR.py lt 0.40 /home/pi/TurtleBorgTypeR.py fd 1 /home/pi/TurtleBorgTypeR.py lt 0.40 /home/pi/TurtleBorgTypeR.py fd 1 /home/pi/TurtleBorgTypeR.py lt 0.40
TurtleBorgTypeRTest.py
#!/usr/bin/env python # coding: Latin-1 import TurtleBorgTypeR as TurtleBorg TurtleBorg.MoveForward(1) TurtleBorg.MoveLeft(0.40) TurtleBorg.MoveForward(1) TurtleBorg.MoveLeft(0.40) TurtleBorg.MoveForward(1) TurtleBorg.MoveLeft(0.40) TurtleBorg.MoveForward(1) TurtleBorg.MoveLeft(0.40)
